Photo by Shubham Dhage / Unsplash
How to use NFTs in Unity?
Non-fungible tokens (NFTs) seem to have exploded out. From art and music to games, these digital assets are selling like Dutch tulips. In this article, we look closely into NFTs and blockchain integrations with our Unity project. I hope you find a way how you can use it to pull NFT images off from any smart contract and put them into your game.
What is an NFT?
An NFT is a digital asset that represents real-world objects like music, art, in-game items and videos. They are bought and sold online, frequently with cryptocurrency, and they are generally encoded with the same underlying software as many cryptos. “Essentially, NFTs create digital scarcity,” says Arry Yu, chair of the Washington Technology Industry Association Cascadia Blockchain Council and managing director of Yellow Umbrella Ventures.
Many NFTs, at least in these early days, were digital creations that already exist in some form elsewhere, like iconic NBA video clips or versions of digital art. For example, the famous digital artist Mike Winklemann, better known as “Beeple,” composed a composition of 5,000 daily drawings to create perhaps the most famous NFT today, “EVERYDAYS: The First 5000 Days,” which sold for $ 69.3 million.
How Is an NFT Different from Cryptocurrency?
NFT stands for a non-convertible token that is built using the same type of programming as a cryptocurrency like Bitcoin or Ethereum, but that’s where the similarity ends. Physical money and cryptocurrencies are “interchangeable” meaning they can be traded or exchanged for each other. They also have the same value — one dollar is always worth another dollar; one Bitcoin is always equal to another Bitcoin. The interchangeability of Crypto makes it a trusted way to carry out transactions on the blockchain. The NFTs behave a bit differently.
How Does an NFT Work?
NFT, just like cryptos, are based on the existing blockchain, which is a distributed public ledger that records transactions. Specifically, NFTs are typically held on the Ethereum blockchain, although other blockchains support them as well. An NFT is created, or “minted” from digital objects that represent both tangible and intangible items, including:
- Art
- GIFs
- Videos and sports highlights
- Collectibles
- Virtual avatars and video game skins
- Music
Basically, NFTs are like physical collectibles, only digital. So, instead of hanging an oil painting on the wall, the buyer gets a digital file containing some content. The buyer also receives exclusive property rights. An NFT can only have one owner at a time. Unique NFT data makes it easy to verify their ownership and transfer tokens between owners. The owner or creator may also store certain information there. For example, artists can sign their works by including their signature in the NFT metadata.
What Are NFTs Used For?
Blockchain and NFT technology give artists and content creators a unique opportunity to monetize their products. For example, artists no longer need to rely on galleries or auction houses to sell their art. Instead, the artist can sell it directly to the consumer as an NFT, which also allows him to keep more profits. In addition, artists can program license fees so they will receive a percentage of the sale each time their art is sold to a new owner.
Time for first implementation
As we have already discussed what NFT is, we can move on to the initial implementation. Let’s start our adventure with the use of blockchain from https://chainsafe.io where we can get access to gaming sdk of theirs for building unity games with blockchain integrations. Say hello to a new gaming economy, in-game NFTs, connecting assets to marketplaces, and cryptowallet login.
Let’s download and install their SDK and start our adventure with SDK. The latest version of the tool can be found at: https://github.com/chainsafe/web3.unity If you’ve successfully downloaded the latest version of the SDK, it’s time to move on to integrating it into Unity.
Fresh Unity project with Chainsafe
Following this, we are going to create a new 3d object a quad in our scene which is going to hold the texture of the nft that we will be loading into our game. Important: You don’t need to load int onto a quad. You can load in into anything, beacause all we are going to be doing is replacing the default materials texture with this. Make sure that the camera can see it :)
Now, we are going to click on the quad and we are just going to add a new script which i am just going to tile “BlockChainInteract”. Now, let’s start working on the code. First, we need two data models. The first one will be used to store metadata from the attribute class, the second one will store NFT metadata from the standard json file. Our data model will look like this:
// Used by metadata class for storing attributes
public class Attributes
{
//The type or name of a given trait
public string trait\_type;
//The value associated with the trait\_type
public string value;
}// Used for storing NFT metadata from standard NFT json files
public class Metadata
{
//List storing attributes of the NFT
public List<Attributes> attributes { get; set; }
//Description of the NFT
public string description { get; set; }
//An external\_url related to the NFT (often a website)
public string external\_url { get; set; }
//image stores the NFTs URI for image NFTs
public string image { get; set; }
//Name of the NFT
public string name { get; set; }
}
Metadata and attributes are part of the standard openc nft documentation as well as standard nft documentation. The most important parameter for as is an image one which stores the URI releated to the actual image stored in blockchain. In addition, the attributes will store the traits and values releated to it, but we are not going to use it. Let’s move into the main script.
We start with four varaibles which define the chain which is polygon, because that’s the chain of the contract will be using in this tutorial. The contract will be associated with which is the project on polygon network. Then, using the ERC721.URI function from our SDK, we can download any data from the blockchain from the indicated network. Here is our code look like:
using Newtonsoft.Json;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.Networking;//Interacting with blockchain
public class BlockChainInteract : MonoBehaviour
{
//The chain to interact with, using Polygon here
public string chain = "polygon";
//The network to interact with (mainnet, testnet)
public string network = "mainnet";
//Contract to interact with, contract below
public string contract = "0xa5f1ea7df861952863df2e8d1312f7305dabf215";
//Token ID to pull from contract
public string tokenId = "54485";
//Used for storing metadata
Metadata metadata;private void Start()
{
//Starts async function to get the NFT image
GetNFTImage();
}async private void GetNFTImage()
{
//Interacts with the Blockchain to find the URI related to that specific token
string URI = await ERC721.URI(chain, network, contract, tokenId);//Perform webrequest to get JSON file from URI
using (UnityWebRequest webRequest = UnityWebRequest.Get(URI))
{
//Sends webrequest
await webRequest.SendWebRequest();
//Gets text from webrequest
string metadataString = webRequest.downloadHandler.text;
//Converts the metadata string to the Metadata object
metadata = JsonConvert.DeserializeObject<Metadata>(metadataString);
}//Performs another web request to collect the image related to the NFT
using (UnityWebRequest webRequest = UnityWebRequestTexture.GetTexture(metadata.image))
{
//Sends webrequest
await webRequest.SendWebRequest();
//Gets the image from the web request and stores it as a texture
Texture texture = DownloadHandlerTexture.GetContent(webRequest);
//Sets the objects main render material to the texture
GetComponent<MeshRenderer>().material.mainTexture = texture;
}
}
}
I hope you liked this simple script and you will use the possibilities offered by blockchain in your projects. Remember that you can download the source code is [here](https://github.com/universityofgames/how-to-use-NFTs-in-Unity).
To help us create more free stuff, consider leaving a donation through Patreon where you can vote for the next asset pack and get access to future packs & articles earlier. Sharing knowledge is our passion. Unfortunately, keeping a team of professionals costs a lot that’s why we are constantly taking on new opportunities.
We’ll be publishing any news on the following outlets:
Thanks for reading!
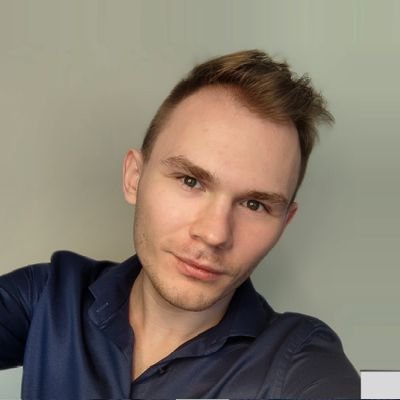
Leszek W. Król
On a daily basis, I accompany companies and institutions in designing strategies and developing new products and services.